Flask Study Note 5
Flask-session with Redis
When building we build applications that handle users, a lot of functionality depends on storing session variables for users. Consider a typical checkout cart: it's quite often that an abandoned cart on any e-commerce website will retain its contents long after a user abandons. Carts sometimes even have their contents persist across devices! To build such functionality, we cannot rely on Flask's default method of storing session variables, which happens via locally stored browser cookies. Instead, we can use a cloud key/value store such as Redis, and leverage a plugin called Flask-Session.
Flask-Session is a Flask plugin which enables the simple integration of a server-side cache leveraging methods such as Redis, Memcached, MongoDB, relational databases, and so forth. Of these choices, Redis is an exceptionally appealing option.
Redis is NoSQL datastore written in C intended to temporarily hold data in memory for users as they blaze mindlessly through your site. Redis was designed for this very purpose, is extremely quick, and is free to use when spinning up a free instance on Redis Labs.
Installation
To get started, we need to install 2 libraries: Flask-Session and Redis:
$ pip3 install flask-session redis
Configuration
Next, we need to import the Redis library with import redis
(we'll get to that in a minute). Next, we need to set the following variables:
- SECRET_KEY: This is not needed any more.
- SESSION_TYPE: Will be set to
SESSION_TYPE=redis
for our purposes. - SESSION_REDIS: The URI of our cloud-hosted Redis instance. Redis URIs are structured a bit uniquely:
redis://:[password]@[host_url]:[port]
.
from flask_session import Session
from redis import Redis
app = Flask(__name__, static_folder="static", static_url_path="/staticfolder")
app.config["SESSION_TYPE"] = "redis"
app.config["SESSION_REDIS"] = Redis("127.0.0.1", 6379, db=7)
Session(app)
Setting a Value
Setting a variable on a session looks a lot like setting values for any old Python dictionary object:
session['key'] = 'value'
Retrieving a Value
With a value saved to our session, we can retrieve and reuse it with .get()
:
session_var_value = session.get('key')
Removing a Value
If the value we've saved has been used and is no longer needed, we can remove variables from our session using .pop()
:
session.pop('key', None)
Use
@app.route("/login", methods=["GET", "POST"], strict_slashes=False)
def login():
if request.method == "GET":
return render_template("login.html")
if request.method == "POST":
user_info = request.form.to_dict()
if user_info.get("username") == "tongye" and user_info.get("pwd") == "123":
session["user"] = user_info.get("username") # <====== THIS LINE =======
return redirect("/")
else:
return render_template("login.html", msg="Wrong username or password")
We are DONE!
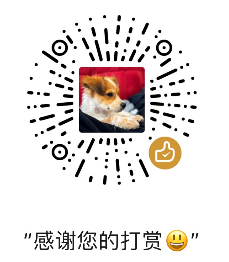