module.exports vs exports
module.exports vs exports
We see module.exports
and exports
often, what are the differences?
Create a file module.js
:
console.log(exports === module.exports);
console.log(exports);
Run in command line:
$ node module.js
true
{}
===
return true, it means they both point to the same null object{}
.
Then, when should I only use module.exports
? and when should I only use exports
? From the perspective of the module developer, there is no difference; the only difference probably is that exports
is 7 characters less than module.exports
. However, from the perspective of module users, there is a difference between these two.
exports.pi = 3.14;
// vs
module.exports.pi = 3.14;
Anonymous function
Assume I, as a module user, want to import a function in my code:
const func = require ('./ module');
then the developer can only use module.exports
to define the function:
module.exports = function () {}
What if the developer uses exports
to define:
exports.func = function () {}
In that case, the user must know the name of the function to use:
const {func} = require ('./ module');
The same goes for replacing functions
with variables
, constants
or other types of data.
Attention object
As I mentioned earlier, module.exports
and exports
point to the same null object {}
and we call it M
. That is to say, no matter if it is exports.pi = 3.14
or module.exports.pi = 3.14
, we are to operate the M objects. As we know, in Node / JavaScript, the objects are alterable and can be modified at will.
Therefore, we can directly assign values to exports
:
exports = 3.14;
But in that case, exports
means nothing at all because it would no longer to points to the object M after variable assignment, so it cannot operate the M object, and cannot control the data which is to be exported by the module, so this operation is wrong and should be avoided.
What about assigning a value to module.exports
?
module.exports = 3.14;
There is no problem, the module exports a value by default, not the M object.
To sum up, in Node.js
module, the exports
is shortcut for referencing module.exports
, if your module is to export an object. It is a pretty much useless object if your module exports any other data type, such as strings, values , Functions, etc., or you have assigned anything to module.exports
.
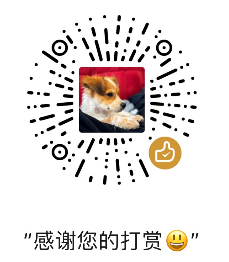